Expression Tree
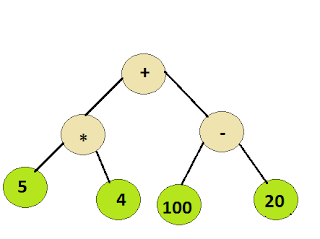
PROBLEM : Given a simple expression tree, which is also a full binary tree consisting of basic binary operators i.e., + , – ,* and / and some integers, Your task is to evaluate the expression tree. You need to complete the function evalTree which takes the root of the tree as its only argument and returns an integer denoting the result obtained by simplifying the expression tree. Input: The first line of input contains an integer T denoting the no of test cases . Then T test cases follow. Each test case contains an integer N denoting the no of nodes. Then in the next line are N space separated values of the nodes of the Binary tree filled in continous way from the left to the right. Output: For each test case output will be the result obtained by simplifying the expression tree. Constraints: 1<=T<=100 2<=N<=50 Example(To be used only for expected Output): Input: 2 7 + * - 5 4 100 20 3 - 4 7 Output: 100 -3 Explanation: For the first test case t